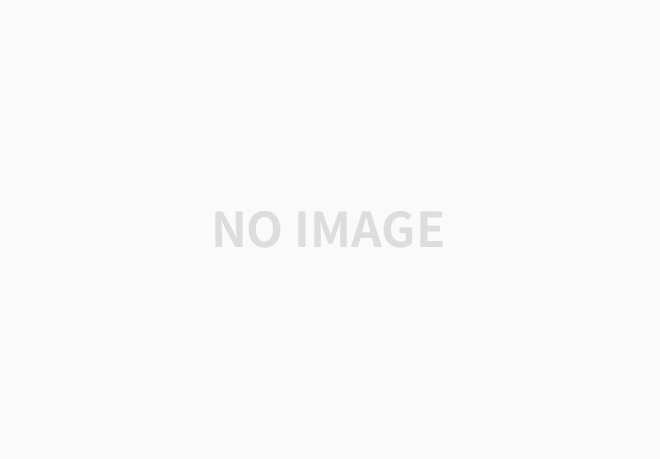
패키지 설치
# Yarn
# redux 관련
yarn add react-redux @reduxjs/toolkit redux-devtools-extension
# 추가 패키지
yarn add react-router-dom sass styled-components
패키지 이름 | 설명 |
react-redux | 리액트에서 redux를 사용할 수 있도록 해주는 컨테이너. redux에 의존한다. |
@reduxjs/toolkit | 리액트에서 리덕스를 좀 더 간결하게 사용할 수 있도록 하는 최신 패키지 |
redux-devtools-extension | 리덕스의 상태를 크롬브라우저 개발자도구에 설치된 확장 기능과 연동할 수 있게 해주는 미들웨어 |
react-router-dom | SPA앱을 만들 때 사용. URL에 따라 실행할 Javascript를 분기한다. |
styled-components | styled component 지원 |
index.js
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import { BrowserRouter } from "react-router-dom";
/** 리덕스 구성을 위한 참조 */
import { Provider } from "react-redux";
import store from "./store";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<Provider store={store}>
<BrowserRouter>
<App />
</BrowserRouter>
</Provider>
);
- Provider는 React-redux 라이브러리에 내장되어 있는, 리액트 앱에 store를 손쉽게 연동할 수 있도록 도와주는 컴포넌트이다.
- BrowserRouter는 HTML5의 history API를 사용해 URL과 UI를 동기화하는 Router이다.
App.js
import React, { memo } from "react";
import { Routes, Route } from "react-router-dom";
import MenuLink from "./components/MenuLink";
import Counter from "./pages/Counter";
const App = memo(() => {
return (
<div>
<h1>13-redux</h1>
<nav>
<MenuLink to="/counter">Counter</MenuLink>
</nav>
<hr />
<Routes>
<Route path="/counter" element={<Counter />} />
</Routes>
</div>
);
});
export default App;
메인에서 Counter 링크를 만들어 클릭하면 카운터 화면을 보여준다.
/slices/CounterSlice.js
import { createSlice } from "@reduxjs/toolkit";
const CounterSlice = createSlice({
name: "CounterSlice",
// 이 모듈이 관리하고자 하는 상태값들을 명시
initialState: {
number: 0,
color: "#000",
},
// 상태값을 갱신하기 위한 함수들을 구현
// 컴포넌트에서 이 함수들을 호출할 때 전달되는 파라미터는 action.payload로 전달된다
// initialState와 동일한 구조의 JSON을 리턴한다
reducers: {
plus: (state, action) => {
const numberValue = state.number + action.payload;
let colorValue = "#000";
if (numberValue > 0) {
colorValue = "#2f77eb";
} else if (numberValue < 0) {
colorValue = "#f60";
}
return { number: numberValue, color: colorValue };
},
minus: (state, action) => {
const numberValue = state.number - action.payload;
let colorValue = "#000";
if (numberValue > 0) {
colorValue = "#2f77eb";
} else if (numberValue < 0) {
colorValue = "#f60";
}
return { number: numberValue, color: colorValue };
},
},
});
// 액션함수들 내보내기
export const { plus, minus } = CounterSlice.actions;
// 리듀서 객체 내보내기
export default CounterSlice.reducer;
Redux Toolkit의 createSlice()는 액션 타입, 액션 생성 함수, 액션별 리듀서, 취합 파일 등을 하나의 파일로 통합할 수 있게 한다.
- createSlice에 선언된 슬라이스 이름을 따라서 리듀서와 액션 생성자, 액션 타입 자동 생성한다.
- createAction, createReducer를 따로 작성할 필요 없다.
리듀서를 만든다.
store.js
import { configureStore } from "@reduxjs/toolkit";
import CounterSlice from "./slices/CounterSlice";
const store = configureStore({
// 개발자가 직접 작성한 Slice 오브젝트들이 명시되어야 한다
reducer: {
CounterSlice: CounterSlice,
},
});
export default store;
만들어놓은 리듀서를 가져온다.
/pages/Counter.js
import React, { memo } from "react";
// 상태값을 로드하기 위한 hook과 action함수를 dispatch함 hook 참조
import { useSelector, useDispatch } from "react-redux";
// Slice에 정의딘 액션함수들 참조
import { plus, minus } from "../slices/CounterSlice";
const Counter = memo(() => {
// hook을 통해 slice가 관리하는 상태값 가져오기
const { number, color } = useSelector((state) => state.CounterSlice);
// dispatch 함수 생성
const dispatch = useDispatch();
return (
<div style={{ display: "flex" }}>
<button onClick={(e) => dispatch(plus(5))}>+5</button>
<h2
style={{
color: color,
margin: "10px",
width: "50px",
textAlign: "center",
}}
>
{number}
</h2>
<button onClick={(e) => dispatch(minus(3))}>-3</button>
</div>
);
});
export default Counter;
카운터 완성
참고 :
https://blog.hwahae.co.kr/all/tech/tech-tech/6946
Redux Toolkit (리덕스 툴킷)은 정말 천덕꾸러기일까?
Redux Toolkit 최근 훅 기반의 API 지원이 가속화되고 React Query, SWR 등 강력한 데이터 패칭과 캐싱 라이브러리를 사용하면서 리덕스 사용이 줄어드는 방향으로 프론트엔드 기술 트렌드가 변화하고 있
blog-wp.hwahae.co.kr
728x90
'Web > react' 카테고리의 다른 글
[리액트] Hooks 알아보기 (0) | 2023.06.08 |
---|---|
[리액트] Redux (0) | 2023.01.06 |
[리액트] export와 export default의 차이 (0) | 2023.01.03 |
[리액트] Props (0) | 2023.01.02 |
[리액트] Components (0) | 2022.12.15 |